View Current Projects
Here's a look at my current projects. As my skillset improves I will update the current projects and add additional projects to the portfolio as well. Please contact me with any positive or constructive feedback.
SoundQuest
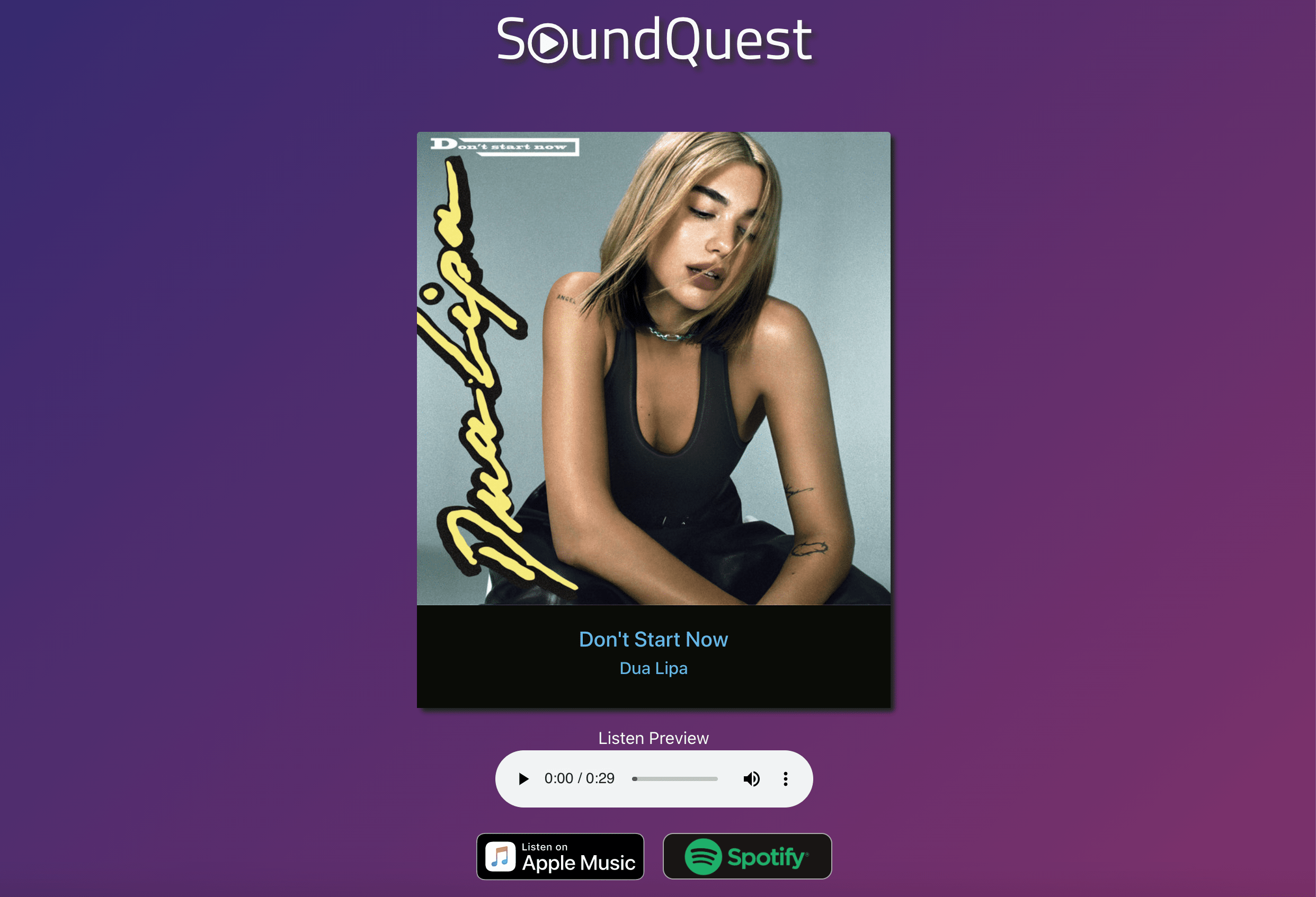
Overview
SoundQuest is a music recognition app built with React JS. The app is similar to Shazam. You can play an actual song or hum a melody and the app will return the song information as well as various media links. There is also basic search capabilities for finding lyrics or song info if you prefer to demo the app in a quiet setting. The app utilizes two separate APIs for fetching data. The AudD API is used for the grunt work for all request while the LastFM API is used for finding additional cover art. Node.JS is used on the backend for a simple solution to store the API Keys. Used Heroku to deploy application.
Technologies Used
- React
- Bootstrap
- SASS
- Node JS
- Express
Challenges
This app brought on a myriad of challenges especially
post-deployment. The biggest problem was handling browser
compatibility issues for both
MediaDevices.getUserMedia()
and
MediaRecorder
methods on mobile devices. Those
methods handle the core functionality of the app, and
depending on the browser and operating system (iOS, Android),
the app may not work at first. I was not able to catch the
issues during development. Because
MediaDevices.getUserMedia()
methods are not allowed on mobile browsers without a secure
HTTPS connection.
Solution
I created conditional statements for validation to determine
the user's browser and device. That way if the user is using
an iOS device, they get an alert to use the safari browser &
instructions to enable
MediaRecorder
via their device settings.
Key Features
- Error Handling for iOS Devices
- CSS/JS animations during recording, loading & success for UX
- External media links for search results (Apple Music, Spotify, YouTube etc.)
- Lyrics Search (Quest Mode) - available for quiet demo
- React Hooks
useState()
- Custom Routes w/ReactRouter
PlayStation Clone Site
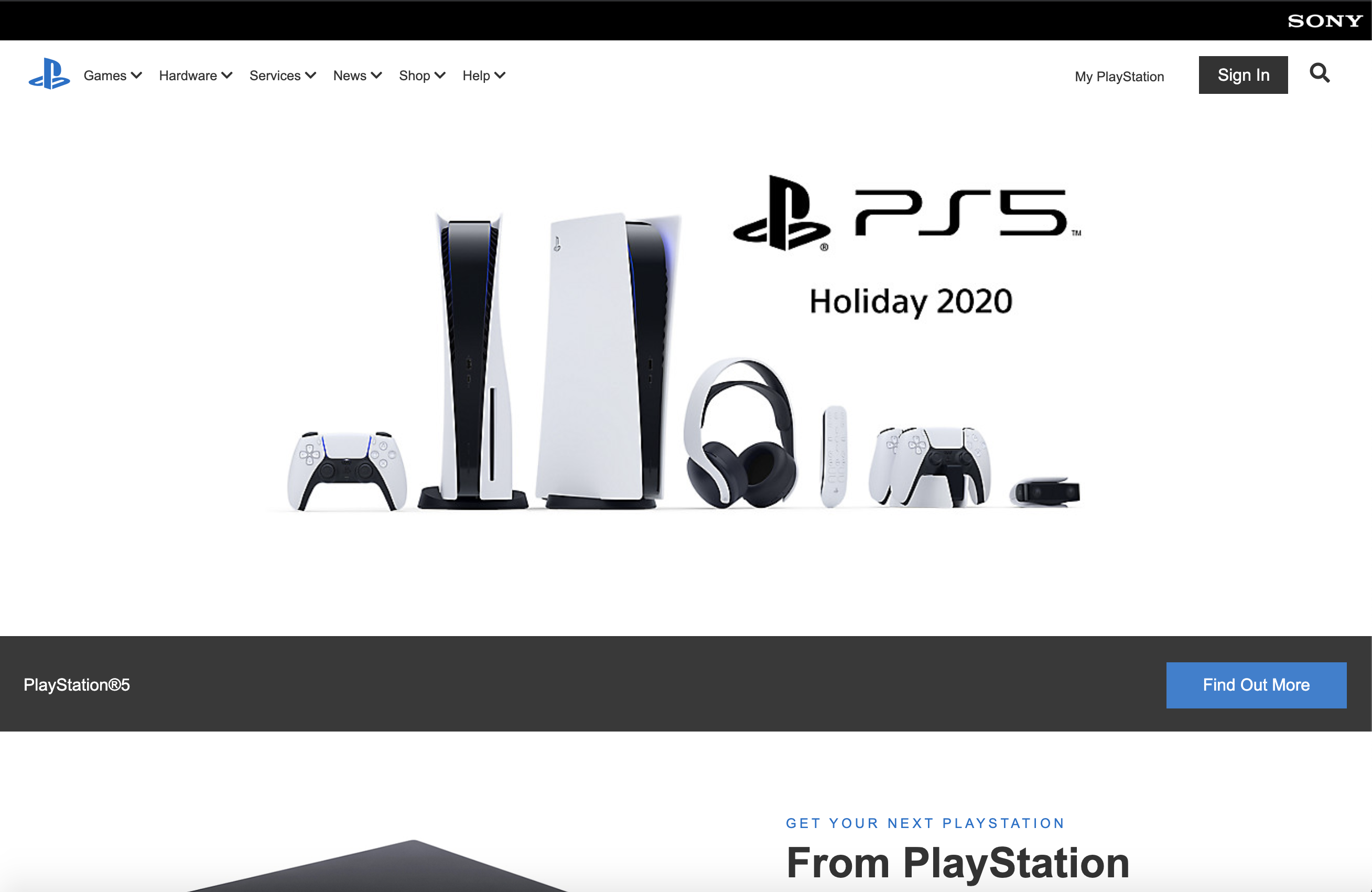
Overview
This clone site was completely built from scratch without any frameworks. This project highlights my abilities to be given a graphic design blueprint and recreate it with code. The website is fully responsive with tablet and mobile breakpoints. All styling was done with SASS and compiled into regular CSS. JavaScript was used for drop down menu animations, as well as scroll events for showcase images and headings. Images were used from the June 2020 version of the official PlayStation website.
Technologies Used
- SASS
- CSS3
- HTML5
- JavaScript
Challenges
This project was relatively difficult to make responsive for mobile and tablet viewports. It was also a challenge to figure out how to use JavaScript to make the menus switch depending on the user's input.
Solution
Reading MDN documentation on CSS fixed positions and overflow
helped me over come the challenges I had with making the
navigation menus responsive across multiple browsers. Also
researching and finding the JavaScript
continue;
keyword when iterating over the clicked
menu items helped fixed the nav menu's functionality.
Key Features
- Search Button Modal
- Scroll event based CSS animations
- Distinct styles for mobile & tablet viewports
JavaScript Calculator App
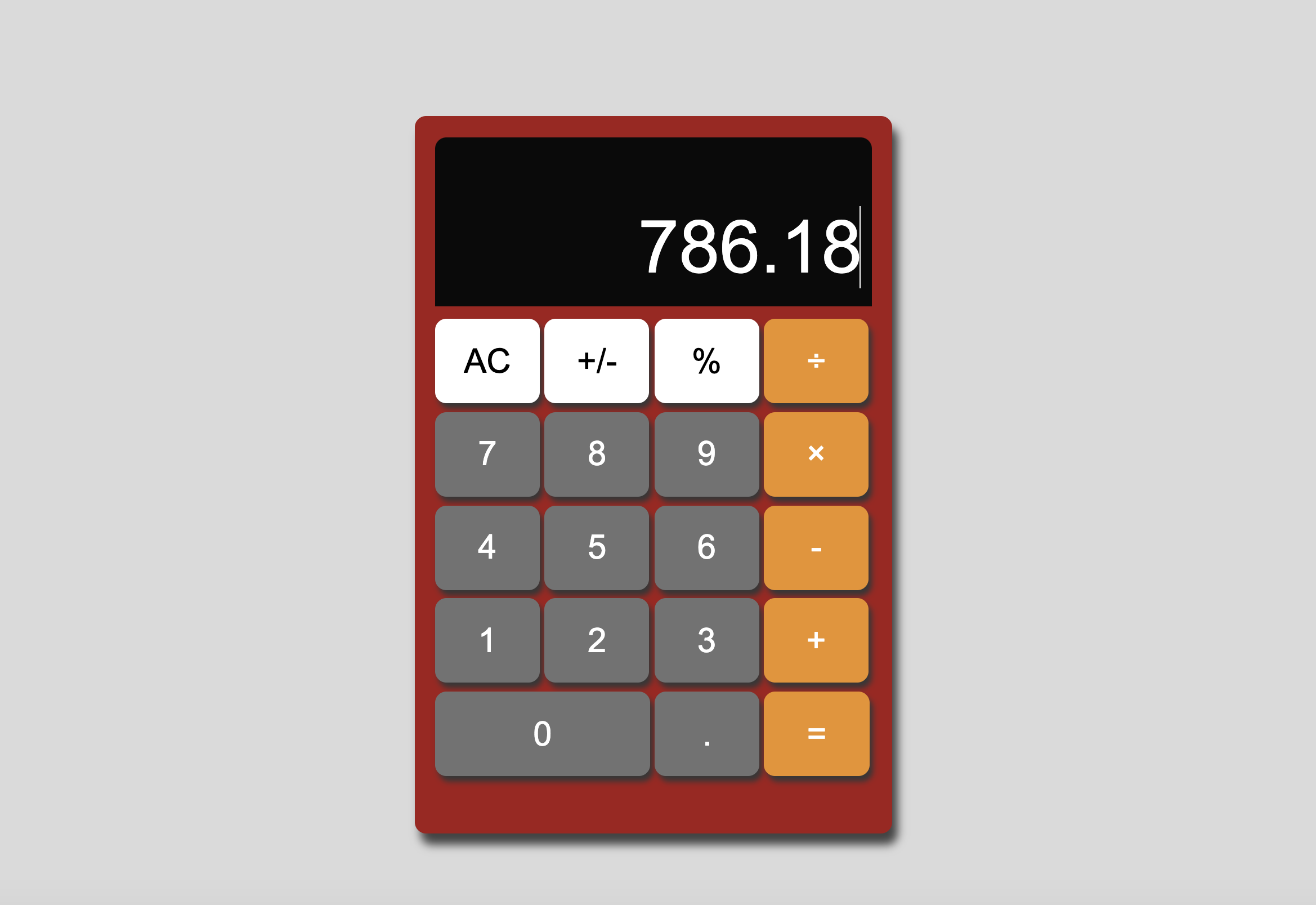
Overview
I picked to build a simple calculator app to demonstrate my knowledge of JavaScript. This application uses a variety of arrays, loops, objects, functions and event handlers to perform the functionality of your typical calculator. If you try to break the app (NaN or Infinity), you will get an error message which is good for user experience. I encourage you to also view the source code via my Github to gain further insight of the functionality.
Technologies Used
- JavaScript
- HTML5
- CSS3
Challenges
The biggest challenge for this project was to resist looking up tutorials online on how to specifically build a calculator. Since this was one of my first JavaScript projects, I wanted to force myself to think like a programmer and look up documentation on specific concepts rather than have everything explained in a video that ultimately I would learn less from.
Solution
As the project progressed I learned how to properly search for resources needed via MDN or Stack Overflow to solve key issues. One of the biggest issues I resolve was creating a validation function where decimals could only be inputted once from the user.
Key Features
- Error handling for NaN or Infinity
- Read-Only inputs to prevent mobile keyboards
JavaScript Weather App
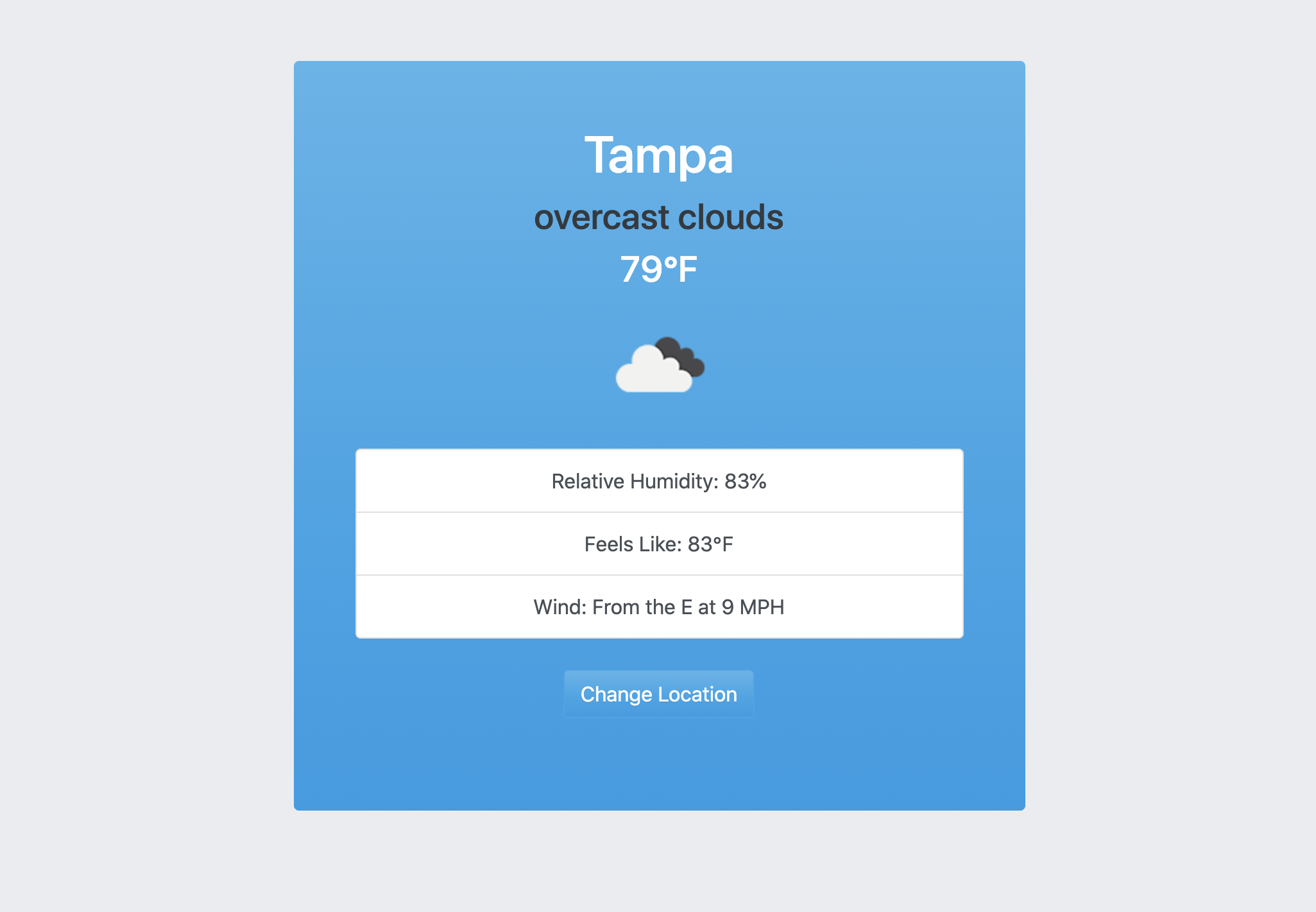
Overview
This project is a simple application where it fetches the current weather of any city based off the user's input. This application utilizes the Open Weather API. I used Brad Traversy's bootstrap theme for the UI, I made some minor styling changes but I wanted to focus on the actual functionality (JS) of the application and not the CSS/UI. The JS code was built from scratch. This project demonstrates my ability of utilizing the Fetch API as well as dealing with Promise based HTTP responses with ES7 async/await.
Technologies Used
- JavaScript
- HTML5
- Bootstrap
Challenges
Comprehending the API documentation as well as strategizing on the best way to utilize the data for the best user experience was the biggest challenge for this project.
Solution
The JSON data response for wind direction was given in degrees, so I created a custom function that would take the wind direction data and convert it into a cardinal direction (N, S, E, W) before showing that data to the user.
Key Features
- Change Location City
- Fetch API
- async/await